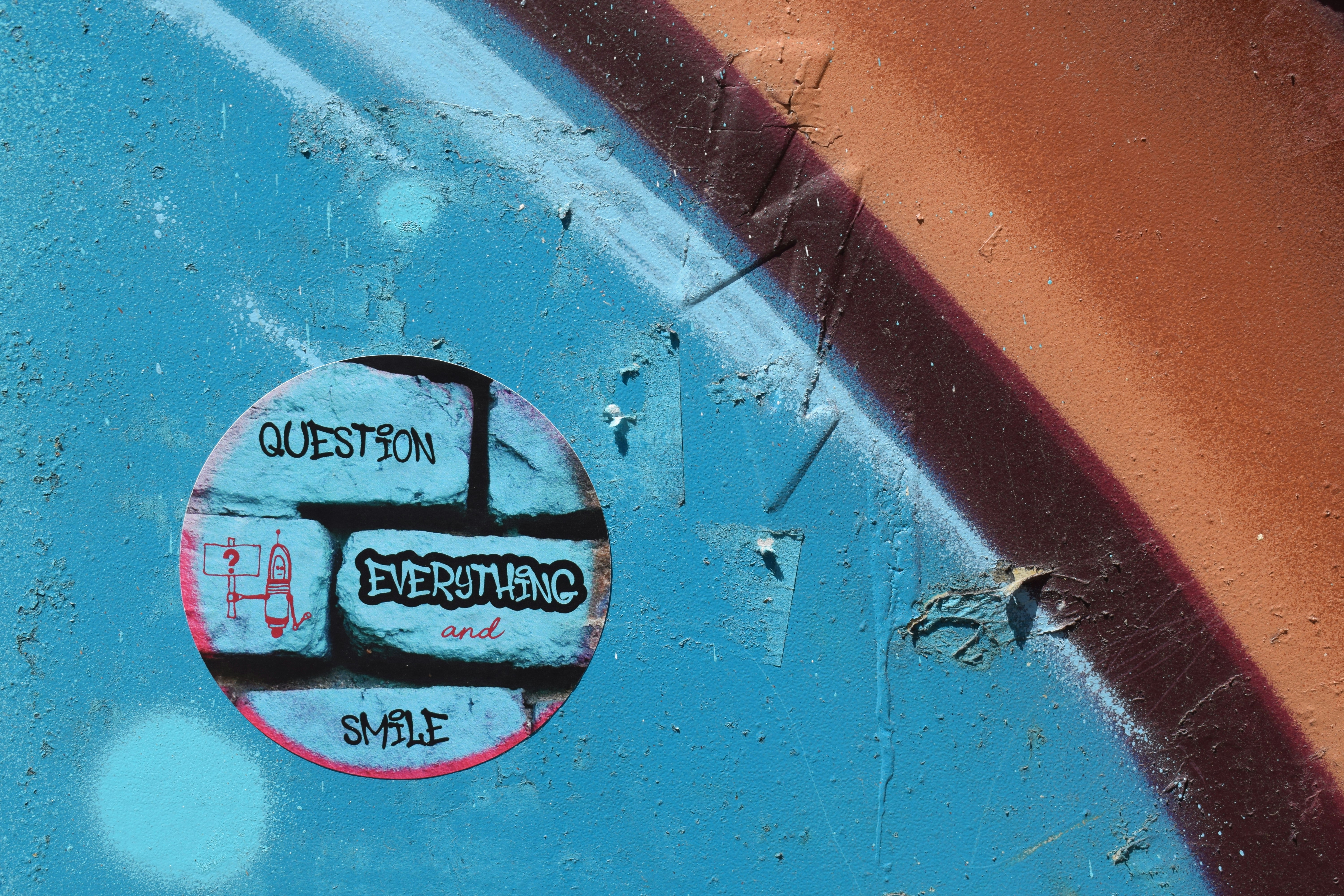
Space Industry Job Interview Warm‑Up: 30 Real Coding & System‑Design Questions
From launch vehicles and satellite constellations to deep-space probes and space tourism, the space industry is experiencing unprecedented growth. Private companies and government agencies alike are seeking professionals skilled in aerospace engineering, software development, communications, mission operations, and robotics to push the boundaries of human exploration and commercial ventures. If you’re pursuing a role in the space sector—whether it’s designing spacecraft systems, developing satellite software, or enabling mission control—thorough interview prep is pivotal.
This blog post tackles 30 real coding & system-design questions you might face when interviewing for a position within the space industry. We’ll also explore why targeted preparation matters, how to showcase domain-specific knowledge, and what traits employers prize—like collaborative problem-solving and resilience under ambitious deadlines. If you’re searching for new space jobs in the UK, check out www.ukspacejobs.co.uk, a specialised resource linking candidates to employers across satellite operations, launch services, and beyond.
Let’s begin with an overview of why rigorous interview readiness is so essential in this challenging and fast-moving field.
1. Why Space Industry Interview Preparation Matters
Space projects can be multi-year endeavours involving high stakes, strict mass/power budgets, and extreme environmental conditions. Interdisciplinary teams bring together mechanical engineers, software developers, systems architects, and scientists from various backgrounds. Here’s why preparation is essential:
Demonstrate Technical Breadth
From orbital mechanics and propulsion to embedded software and radio communications, roles in the space sector often touch on multiple disciplines.
Employers will test whether you can integrate knowledge from hardware, software, and operational constraints.
Show Systemic Thinking
Space missions require reliability and robustness at every level, from spacecraft avionics to ground control.
Interviews often probe your ability to handle end-to-end system design: data flows, power budgets, fault tolerance, safety margins, and more.
Highlight Reliability & Safety
Once launched, satellites or spacecraft can’t be physically repaired easily. Missions must succeed within tight constraints.
Employers want you to demonstrate an approach to testing, verification, and risk management that ensures minimal in-flight failures.
Emphasise Team Collaboration
Designing rockets or satellites is rarely a solo activity. Cross-functional collaboration with mission planners, payload specialists, communication experts, and flight dynamics is standard.
Show how you’ve worked in diverse teams, integrated feedback, and managed complex interfaces.
Reflect Adaptability to Cutting-Edge Tech
The space industry is witnessing new materials, reusable rockets, mega-constellations, and novel deep-space missions.
Employers value candidates who can quickly adopt new technologies or processes as the field evolves.
With strong preparation, you’ll be poised to address technical and soft skill questions, proving you’re ready to contribute to challenging missions. Next, we’ll walk through 15 coding questions frequently posed in space-related roles.
2. 15 Real Coding Interview Questions
Coding Question 1: Embedded System for Satellite Control
Question: Write embedded C code to toggle a GPIO pin that powers a satellite subsystem on/off, ensuring it can’t exceed maximum duty cycle for thermal reasons.
What to focus on:
Setting up microcontroller registers,
Implementing a timer-based approach to limit on-time,
Handling fault conditions (e.g., subsystem overheats).
Coding Question 2: Telemetry Parsing
Question: You receive raw telemetry packets from a satellite (e.g., temperature, voltage, status flags). Demonstrate how you’d parse and store these in a data structure.
What to focus on:
Handling packet headers, lengths, checksums,
Converting raw bytes to engineering units (floats, ints),
Dealing with potential partial packets or corrupted data.
Coding Question 3: Command & Data Handling
Question: Implement code that manages incoming commands from ground control, verifying them (CRC) and executing actions if valid.
What to focus on:
Checking CRC or hash to ensure message integrity,
A command dispatcher pattern for different opcodes,
Logging executed commands for replay or post-mission analysis.
Coding Question 4: RTOS Scheduling
Question: Show how you’d structure tasks in a real-time OS (RTOS) for a cubesat’s flight software: one for sensor updates, one for comms, one for payload operations.
What to focus on:
Priority assignment,
Using semaphores or queues for data exchange,
Ensuring critical tasks have enough CPU time.
Coding Question 5: Reliable Data Storage in Orbit
Question: Code a function that writes sensor logs to flash memory with wear leveling, ensuring minimal corruption if power is lost.
What to focus on:
Partitioning flash blocks,
Using a simple journaling or log-structured approach,
Handling partial writes and verifying data on restart.
Coding Question 6: C++ for Satellite Simulation
Question: Write a snippet that simulates orbit propagation (basic two-body) and logs the satellite’s position at each timestep.
What to focus on:
Newtonian gravitational formula,
Euler integration or more advanced integrators,
Data struct for (x,y,z) or using vector libraries.
Coding Question 7: Command-Line Tool for Ground Station
Question: Implement a Python CLI that sends encoded commands via TCP to your satellite’s server emulator, logs replies, and exits on user request.
What to focus on:
Socket communication,
Parsing user inputs,
Handling timeouts or unexpected server disconnects.
Coding Question 8: Watchdog & Health Monitor
Question: In a satellite’s flight software, code a watchdog timer that reboots the system if main loop is unresponsive for 2 seconds.
What to focus on:
Setting up a hardware watchdog,
Periodic “kick” from main loop,
If no kick received, system triggers reset, logs reason if possible.
Coding Question 9: Image Compression on Board
Question: Show how you’d compress raw camera images in C/C++ to JPEG or a custom scheme before downlink, given limited CPU.
What to focus on:
Possibly using a lightweight library or custom DCT-based approach,
Managing memory constraints (chunks),
Handling partial transmissions or progressive compression.
Coding Question 10: ECS (Environmental Control System) Logic
Question: Code a function that reads multiple temperature sensors, compares with setpoints, and toggles heaters or fans accordingly.
What to focus on:
Handling multiple sensor inputs,
Minimising hysteresis (avoid frequent toggles),
Logging expansions if temperature drifts out of range.
Coding Question 11: CRC vs. Checksum
Question: Implement a 16-bit CRC function in C, then compare it with a simpler additive checksum function.
What to focus on:
CRC polynomial usage,
Step-by-step or table-based approach,
Pros/cons in terms of error detection coverage.
Coding Question 12: Ground Station GUI
Question: Write a basic Python/Qt code snippet that displays real-time orbit telemetry in a graph, updating every second.
What to focus on:
Timer-based updates (QTimer),
Thread-safe queue or direct reading from a socket,
Handling partial data gracefully.
Coding Question 13: FPGA or HDL for Payload Data
Question: Outline a Verilog module that buffers and serialises payload data at a defined bit rate for transmission.
What to focus on:
FIFO for data buffering,
State machine for shifting bits out,
Clock domain crossing if needed.
Coding Question 14: Python Script for Mission Analysis
Question: Demonstrate how you’d compute satellite coverage times over a specific ground station using orbital elements (TLE).
What to focus on:
Reading TLE, using SGP4 or a library for orbit propagation,
Calculating line-of-sight geometry with Earth’s horizon,
Output time windows for coverage.
Coding Question 15: Caching Telecommands
Question: Write code that caches telecommands from ground if the satellite is busy, then executes them once the system is idle.
What to focus on:
Circular buffer or queue data structure,
Checking system state before dequeuing next command,
Handling priority commands that skip queue.
These coding questions evaluate your proficiency in embedded software, communication protocols, simulation, data handling, and more—skills crucial to space missions. Next, let’s examine 15 system & architecture design scenarios relevant to the space domain.
3. 15 System & Architecture Design Questions
Design Question 1: Satellite Avionics Architecture
Scenario: Propose a high-level architecture for a small satellite’s avionics: flight computer, power system, attitude control, payload.
Key Points to Discuss:
Data buses (CAN, I2C, SpaceWire),
Redundancy or single-string approach,
Processing modules, memory, watchdog strategies.
Design Question 2: Ground Segment & Operations
Scenario: You have a remote ground station controlling a low Earth orbit (LEO) satellite. Outline how you’d manage scheduling passes, commanding the satellite, and storing telemetry.
Key Points to Discuss:
Pass prediction, TLE usage,
Automated command upload windows,
Database for long-term telemetry archiving, alerts on anomalies.
Design Question 3: Launch Vehicle GNC (Guidance, Navigation, Control)
Scenario: Design a system for a small rocket’s flight computer that handles sensor fusion (IMU, GPS), guidance profiles, and stage separation logic.
Key Points to Discuss:
Control law for pitch/yaw/roll,
State machine for staging,
Handling sensor dropout or noise under high acceleration.
Design Question 4: Deep-Space Probe Communication
Scenario: A probe orbiting Mars must transmit data to Earth. Explain how you’d design a robust comm link (antenna selection, data rates, error correction).
Key Points to Discuss:
Constraints: large distance, DSN usage,
Link budgeting, forward error correction (Reed-Solomon, LDPC),
Interplanetary signal delay, store-and-forward approach.
Design Question 5: Satellite Constellation Design
Scenario: You’re tasked with designing a 50-satellite constellation providing Earth imaging. Outline how you handle orbit selection, coverage, inter-satellite links, ground segment integration.
Key Points to Discuss:
Orbital parameters (sun-synchronous, inclination),
Constellation geometry (Walker delta?),
Data relay approach (optical inter-sat links, ground station network).
Design Question 6: Thermal Control System
Scenario: Your spacecraft has multiple high-power components generating heat in low Earth orbit. Propose how to manage heat flow, conduction, and radiation.
Key Points to Discuss:
Heat pipes or conduction paths,
Radiator panels for IR emission,
Active vs. passive thermal control, sensor-based loops.
Design Question 7: Radiation-Hardened Electronics
Scenario: Outline the architecture for a mission going beyond Earth’s magnetosphere, focusing on component selection and data protection from cosmic radiation.
Key Points to Discuss:
Rad-hard or rad-tolerant components,
Error-correcting codes in memory, triple modular redundancy,
Testing in heavy-ion or proton beams if possible.
Design Question 8: Fault Tolerant Design
Scenario: A long-duration lunar lander mission needs fault-tolerant avionics. Explain your approach to redundancy, cross-strapping, and safe modes.
Key Points to Discuss:
Master-slave flight computers,
Cross-strapped communication buses,
Autonomous safe mode triggers if anomalies occur.
Design Question 9: Mission Ops Software
Scenario: Plan a software system that schedules satellite observations, tracks orbital events, and controls payload tasks automatically.
Key Points to Discuss:
Event-driven architecture (sunrise, eclipse entry, ground pass),
Payload scheduling constraints (thermal, power),
Automatic conflict resolution or operator override.
Design Question 10: Real-Time Earth Observation Pipeline
Scenario: A satellite captures high-res images, processes them on board, and transmits only relevant data due to bandwidth limits. Propose the onboard processing architecture.
Key Points to Discuss:
Edge AI for image segmentation/compression,
Onboard storage, priority queue for transmission,
Minimising CPU/GPU usage under power constraints.
Design Question 11: Space Debris Collision Avoidance
Scenario: Your satellite must dodge debris. Describe how you integrate orbit predictions (from TLE updates) and plan manoeuvres.
Key Points to Discuss:
Collision probability calculations,
Autonomous vs. ground-commanded manoeuvre triggers,
Impact on mission schedule, fuel consumption.
Design Question 12: CubeSat Deployment & Control
Scenario: You’re building a 3U CubeSat with limited power. Summarise your design for power generation (solar), battery management, comms, and attitude control.
Key Points to Discuss:
Deployable solar panels, MPPT (maximum power point tracking),
Reaction wheels or magnetorquers for attitude,
Minimal telemetry link (UHF or S-band).
Design Question 13: Multi-Sensor Satellite Payload
Scenario: A single satellite carries both hyperspectral and radar sensors. Outline how you’d share onboard processing resources, data buses, and memory.
Key Points to Discuss:
Scheduling sensor operation to avoid resource contention,
In-flight reconfiguration to handle variable data rates,
Possibly prioritising data from one sensor if power limited.
Design Question 14: Rover-Lander Communication
Scenario: A Mars rover must communicate with an orbiting relay satellite. Propose how you handle line-of-sight disruptions and store-and-forward data.
Key Points to Discuss:
Rover transmissions only when orbiter is overhead,
Reliable link with forward error correction,
Caching data onboard rover, flushing when orbiter pass occurs.
Design Question 15: Launch Support & Telemetry
Scenario: You manage the telemetry system for a rocket launch. Outline how ground stations track rocket flight, collect sensor data, and handle potential booster recovery.
Key Points to Discuss:
Network of tracking antennas (C-band, S-band),
High-rate data link for telemetry (flight path, engine parameters),
Booster location tracking post-separation, recovery procedure.
These system design challenges reveal your grasp of space mission architecture, from orbit planning and thermal management to communication protocols and fault tolerance. Finally, let’s wrap up with interview tips for success in the space sector.
4. Tips for Conquering Space Industry Job Interviews
Master the Fundamentals
Revisit orbital mechanics (Kepler’s laws, TLE usage), RF communications, aerospace structures, or embedded systems—depending on your focus.
Show your ability to apply these fundamentals to real missions.
Familiarise with Standards & Protocols
The space domain often relies on CCSDS protocols, CAN for spacecraft bus, SpaceWire, or custom serial links.
If you’re in software, also know how ground station software (like NASA GMSEC or open-source) interacts with satellites.
Highlight Mission Phases & Constraints
Many questions revolve around handling different mission phases: launch, LEOP (Launch & Early Orbit Phase), cruise, entry, landing.
Employers want to see awareness of resource constraints, radiation exposure, or limited comm windows.
Show Testing & Verification Rigor
Space hardware and software undergo extensive test: HIL (hardware in the loop), vibe tests, thermal vac, etc.
Mention how you approach test automation, QA for flight code, or prototyping for risk reduction.
Demonstrate Team Collaboration
Satellite or rocket programs are massive undertakings with cross-functional teams.
Offer examples of integrating mechanical feedback, fulfilling customer (government or commercial) requirements, or handling iterative design reviews.
Ask About Missions & Timelines
If the company has specific missions or timelines, you might ask how they handle scheduling, regulatory approvals, or partnerships with launch providers.
This shows genuine interest in their overarching mission objectives.
Address Reliability & Redundancy
Single-point failures in space can doom a mission. Outline how you embed redundancy or degrade gracefully if a subsystem fails.
Emphasise risk analyses, FMEAs (Failure Modes & Effects Analysis).
Leverage Real Experience
If you’ve done hobby rocketry, participated in a cubesat project, or contributed to a space robotics challenge, share those experiences.
Hands-on or competition-based exposure can weigh heavily in your favour.
Stay Informed on Space Trends
LEO mega-constellations, reusability, small launchers, cislunar missions—space is evolving fast.
Interviews might include “What do you think of Starship?” or “How do you see smallsat tech impacting ground networks?”
Keep an Enthusiastic Mindset
Space is a domain of passion—employers appreciate genuine excitement for exploration and innovation.
Present yourself as a curious, dedicated problem-solver who embraces the big challenges.
By combining robust domain knowledge, proven engineering or software skills, and a collaborative ethos, you’ll convey readiness for the complex, exhilarating tasks the space industry presents.
<a name="section5"></a>
5. Final Thoughts
Space exploration merges engineering precision, scientific discovery, and bold ambition. Whether you aim to build satellites, design rocket systems, or develop the software that orchestrates deep-space missions, your interviewers will seek signs of technical mastery and practical problem-solving. By revisiting the 30 questions here—covering both coding and system-level design—you’ll sharpen your readiness to address topics from orbital mechanics and flight software to mission ops and ground segment architecture.
Just as important as knowledge is your capacity to innovate, collaborate, and adapt to evolving frontiers. If you’re now primed to find your place in this dynamic field, www.ukspacejobs.co.uk offers a gateway to space-industry roles across the UK—helping you connect with companies that develop satellites, launch vehicles, rovers, and more.
With thorough preparation, a sense of curiosity, and a passion for pushing boundaries, you’ll be well on your way to contributing to humankind’s next great leaps into low-Earth orbit, lunar missions, or even deep-space exploration—all while forging a remarkable career trajectory in the final frontier.